Interfacing Sound Sensor with Arduino
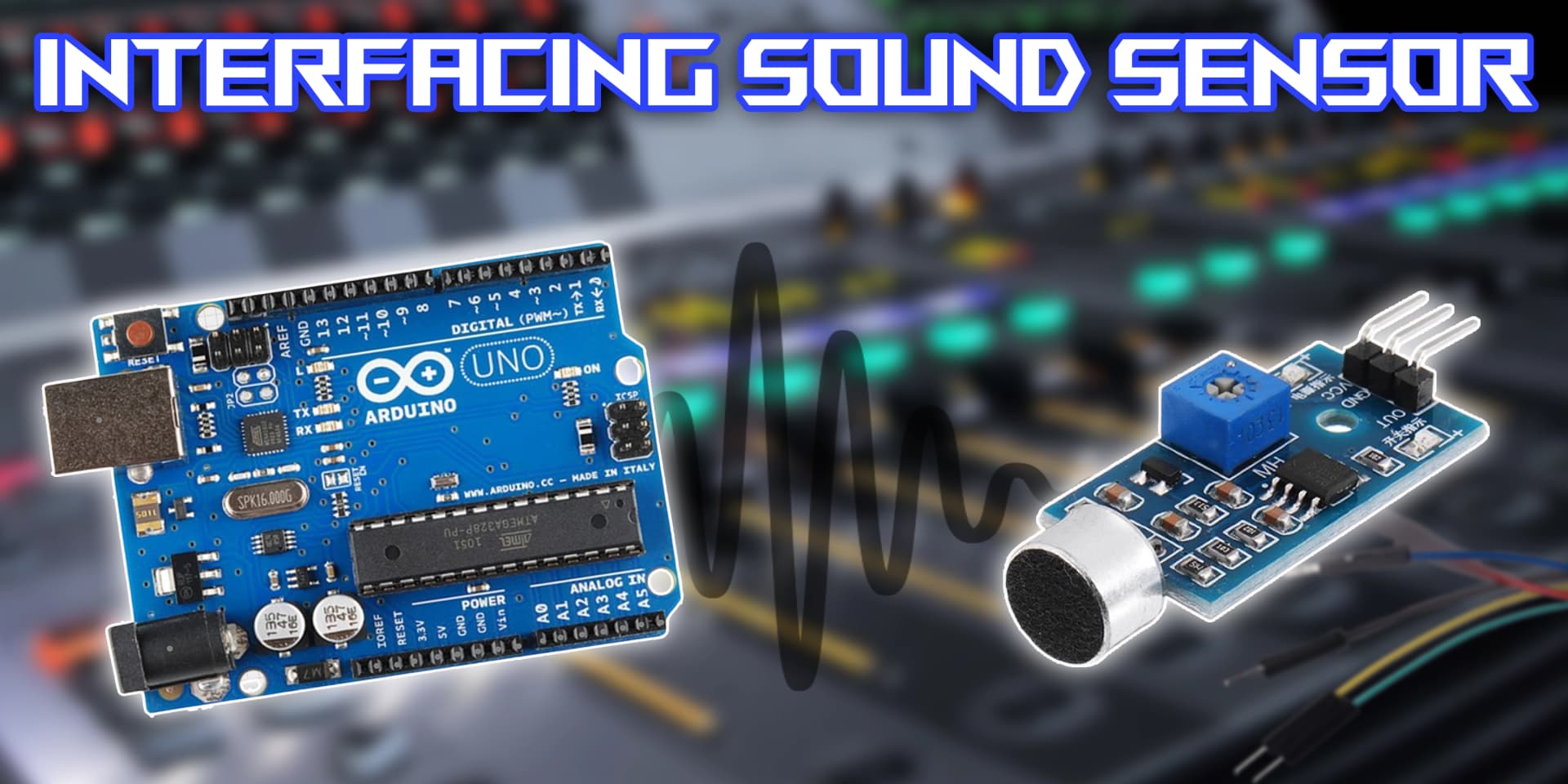
Sound Sensor
A sound sensor detects sound or noise in the environment and converts it into an electrical signal that can be read by an Arduino. It usually contains a microphone and a built-in amplifier to process sound waves and output a signal that can be analyzed by the Arduino. The sensor is widely used in sound detection systems, security alarms, and smart home applications.
How Sound Sensor Works
The sound sensor works by capturing sound waves from the environment using a microphone. These sound waves are then converted into electrical signals. The sensor typically has an analog output that corresponds to the loudness of the sound and a digital output that can be used to trigger events when a certain threshold is exceeded.
Types of Sound Sensors
Analog Sound Sensor
- The sensor detects sound waves and produces an analog voltage signal.
- The Arduino can measure this voltage to determine the loudness of the sound.
Digital Sound Sensor
- The sensor outputs a digital signal when the sound level surpasses the threshold.
- The Arduino reads this signal to detect whether sound has been detected or not.
Requirements
Pin Configuration of Sound Sensor
Sound Sensor Configuration
- VCC: Connect to +5V on Arduino.
- GND: Connect to GND on Arduino.
- Analog Output: Connect to an analog input pin (e.g., A0) for analog signal.
- Digital Output: Connect to a digital input pin (e.g., D2) for digital signal.
Wiring the Sound Sensor to Arduino
To wire the sound sensor to the Arduino, connect the VCC pin to +5V and the GND pin to ground. The analog output (for measuring intensity) should be connected to an analog input pin (e.g., A0), while the digital output (for detecting sound/noise presence) should be connected to a digital pin (e.g., D2) on the Arduino.
Algorithm
Initialize Components
- Connect the VCC and GND pins of the sound sensor to +5V and GND on the Arduino.
- Connect the analog output pin (A0) or digital output pin (D2) to the appropriate Arduino pin.
Write the Code
- In the setup() function, initialize the serial monitor and set the analog or digital pin as input.
- In the loop() function, read the sensor value from the analog or digital pin.
Process the Sound Data
- If using an analog sensor, use the Arduino's analogRead() function to measure the sound intensity.
- If using a digital sensor, monitor the signal for HIGH/LOW to detect sound presence.
Display Sound Levels
- For analog sensors, output the sound intensity on the serial monitor.
- For digital sensors, output whether sound is detected (HIGH) or not (LOW).
Trigger Events Based on Sound
- Use an if statement to trigger actions based on the sound levels (e.g., turn on a light or sound an alarm when a certain threshold is reached).
1// Arduino Sound Sensor Interface
2// This code reads the analog value from a sound sensor and displays it on the Serial Monitor
3
4const int soundPin = A0; // Sound sensor connected to analog pin A0
5int soundValue = 0; // Variable to store sound level
6
7void setup() {
8 Serial.begin(9600); // Start serial communication
9 pinMode(soundPin, INPUT); // Set the sound sensor pin as input
10 Serial.println(\"Sound Sensor Ready - Speak or clap near the sensor\");
11}
12
13void loop() {
14 soundValue = analogRead(soundPin); // Read the sound level
15 Serial.print(\"Sound Level: \");
16 Serial.println(soundValue);
17
18 if (soundValue > 600) { // Threshold for detecting loud sound (adjust as needed)
19 Serial.println(\"Loud sound detected!\");
20 }
21
22 delay(100); // Delay to prevent flooding the Serial Monitor
23}
Applications of Sound Sensors
- Sound-based security systems
- Noise level monitoring
- Voice-activated systems
- Sound detection for robots and automation
- Sound-triggered alarms
- Smart home devices (e.g., voice assistants)
Conclusion
Interfacing a sound sensor with Arduino is a simple and effective way to add sound detection capabilities to your projects. By using analog or digital sound sensors, you can monitor sound levels, detect noise events, and trigger actions based on sound. This project can be applied to various applications, including security systems, sound detection, and smart home automation.