Interfacing MQ-7 CO Carbon Monoxide Coal Gas Sensor Module with Arduino
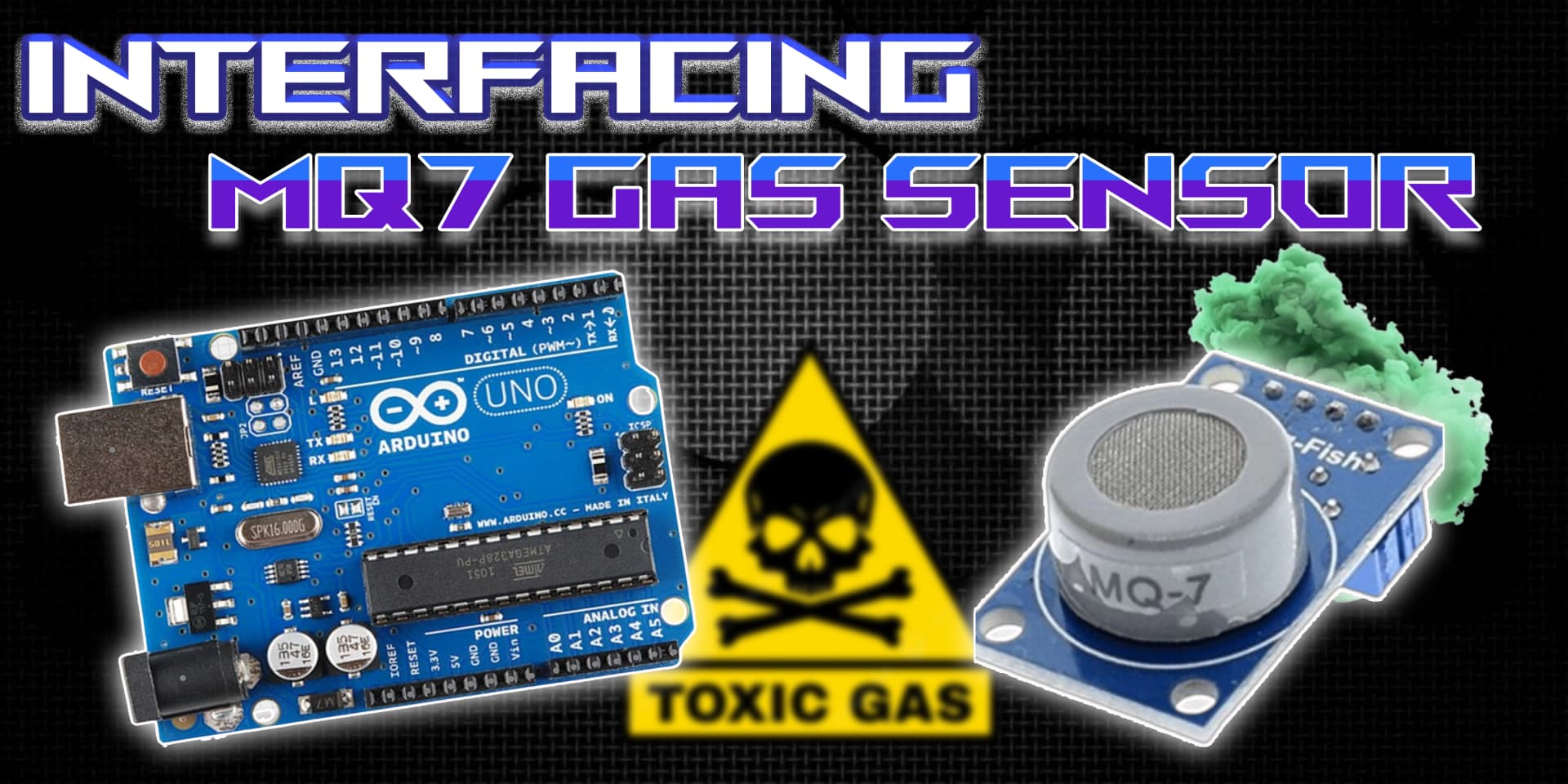
MQ-7 CO Gas Sensor Module
The MQ-7 is a popular gas sensor module used for detecting Carbon Monoxide (CO) and coal gas in the air. It's widely used in safety devices and air quality monitoring systems to prevent exposure to toxic gases.
Working Principle of MQ-7 Sensor
The MQ-7 sensor uses a sensitive layer of SnO2 (tin dioxide), which changes its resistance in the presence of carbon monoxide. The change in resistance alters the voltage across the sensor, which can be measured by an analog input on Arduino.
Features of MQ-7 Sensor Module
Gas Detection
- Contains a built-in heating element to prepare the sensing material.
- Detects variations in gas concentration and converts it to voltage.
- Can operate in both analog and digital output modes.
High Sensitivity & Fast Response
- Heats up in cycles for stable sensing.
- Outputs accurate voltage variations based on gas concentration.
- Responds within seconds to gas presence.
Requirements
1. Arduino Board
2. MQ-7 CO Gas Sensor Module
3. Breadboard
4. Jumper Wires
Pin Configuration of MQ-7 Sensor
MQ-7 Sensor Module
- VCC: Connect to +5V on Arduino.
- GND: Connect to GND on Arduino.
- DO: Digital output pin – goes HIGH when gas is detected.
- AO: Analog output pin – provides variable voltage based on gas level.
Wiring the MQ-7 Sensor to Arduino
To connect the MQ-7 to Arduino: link the VCC and GND pins to 5V and GND. Connect the AO pin to an analog input on the Arduino for reading gas levels. Optionally, connect DO to a digital pin to monitor threshold-based detection.
Algorithm
Initialize Components
- Connect the sensor's VCC to 5V and GND to GND on the Arduino.
- Connect the AO pin to an analog input (e.g., A0).
- Optionally, connect DO to a digital pin for threshold detection.
Write the Code
- Set up the analog pin as INPUT in the setup() function.
- In loop(), read the analog value from the AO pin.
- Map the readings to indicate gas concentration levels.
Display or Alert
- Print the gas levels on the serial monitor.
- Trigger LEDs or buzzers when CO level exceeds a safe limit.
Test the System
- Upload the code to Arduino.
- Expose the sensor to a small source of CO (like smoke from incense) and observe readings.
Arduino Code
1// Pin Definitions
2const int analogPin = A0; // Analog output pin from MQ-7
3const int digitalPin = 2; // Digital output pin from MQ-7 (optional)
4int coLevel = 0; // Variable to store gas value
5
6void setup() {
7 Serial.begin(9600); // Initialize serial monitor
8 pinMode(digitalPin, INPUT); // Set digital pin as input
9 Serial.println("MQ-7 CO Gas Sensor Initialized...");
10}
11
12void loop() {
13 coLevel = analogRead(analogPin); // Read analog gas level
14 int thresholdReached = digitalRead(digitalPin); // Read digital pin
15
16 Serial.print("Analog CO Level: ");
17 Serial.println(coLevel); // Show gas level
18
19 if (thresholdReached == LOW) {
20 Serial.println(" High CO Concentration Detected!");
21 } else {
22 Serial.println(" CO Levels Normal.");
23 }
24
25 delay(1000); // 1 second delay
26}
27
Applications of MQ-7 CO Gas Sensor
- Home gas leak detectors
- Portable gas monitors
- Air quality monitoring systems
- Smart ventilation systems
- Industrial safety applications
- Automotive CO detection
Conclusion
Interfacing the MQ-7 Carbon Monoxide Sensor with Arduino is essential for developing reliable gas detection systems. It helps improve safety by identifying dangerous gas levels in real-time using simple wiring and code.