Interfacing LED and Push Button
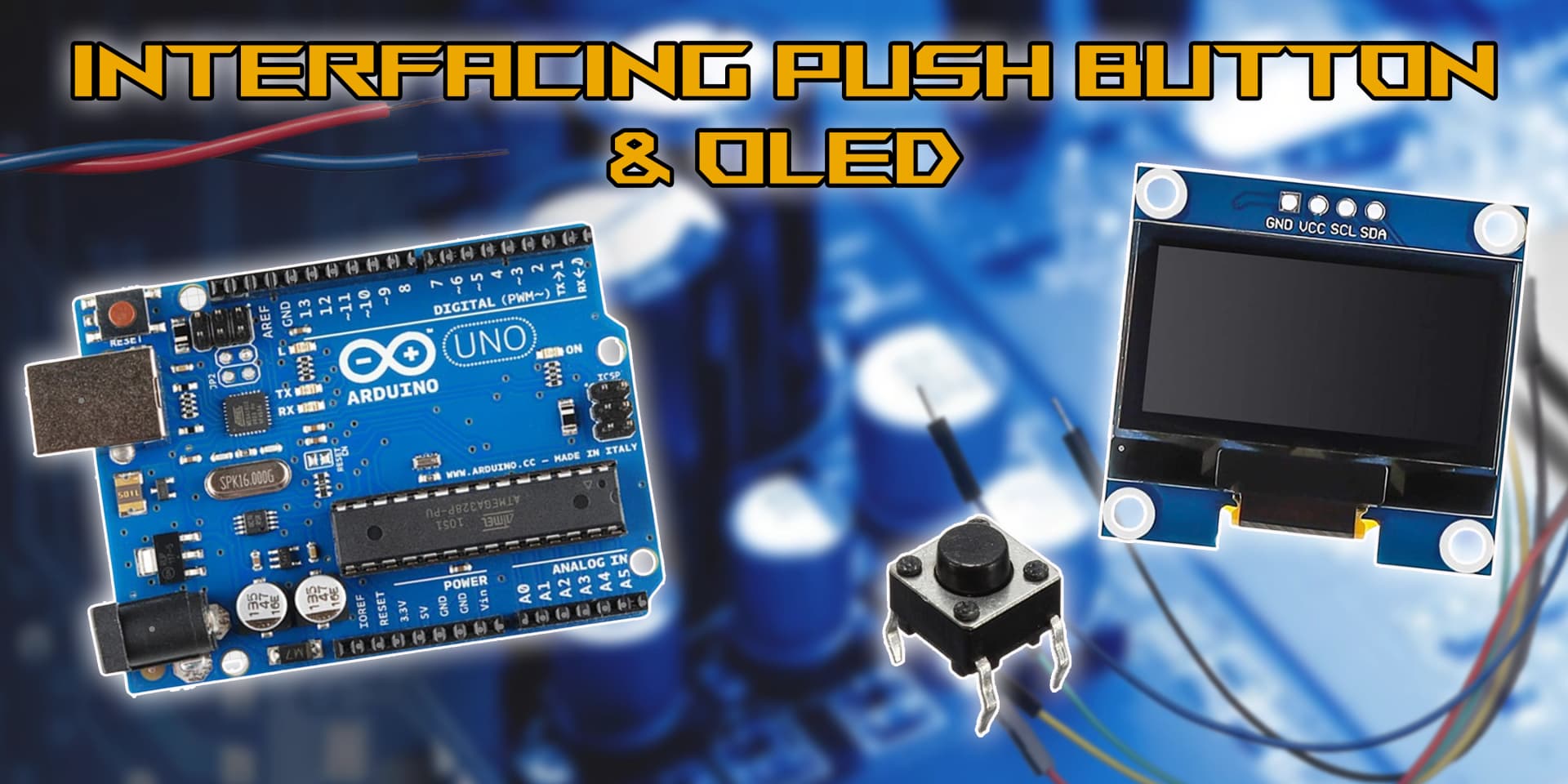
Written By - Tanya Sharma
Have you ever wished to play "light on, light off" with your Arduino? Well, you can accomplish that with just an LED and a push button! Imagine your LED blinking as if to say "hello" as you click a button. Are you prepared to explore this interactive magic?
Have you ever wished to play "light on, light off" with your Arduino? Well, you can accomplish that with just an LED and a push button! Imagine your LED blinking as if to say "hello" as you click a button. Are you prepared to explore this interactive magic?
How does this operate, then? The Arduino receives the signal to illuminate the LED when you press the button! It appears as though the LED is blinking in response to a secret message that your button is sending. Interested in learning how to do this enjoyable project? Let's begin!
An overview of LED and Push Button
When electricity passes through an LED (Light Emitting Diode), a tiny, energy-efficient light source, light is released. LEDs are adaptable for electronics projects since they are available in a variety of colors and sizes. They are extensively employed in lighting, displays, and indicators. They are ideal for embedded systems and do-it-yourself projects due to their long lifespan and low power consumption.A push button is a basic switch that, when depressed, regulates an electrical circuit. It has the power to establish or break the link, which would cause a project activity. In electronics, push buttons are frequently used for human inputs like initiating or terminating operations. Because they enable users to operate devices with a single press, they are crucial in interactive systems. A push button is a basic switch that, when depressed, regulates an electrical circuit. It has the power to establish or break the link, which would cause a project activity. In electronics, push buttons are frequently used for human inputs like initiating or terminating operations. Because they enable users to operate devices with a single press, they are crucial in interactive systems.
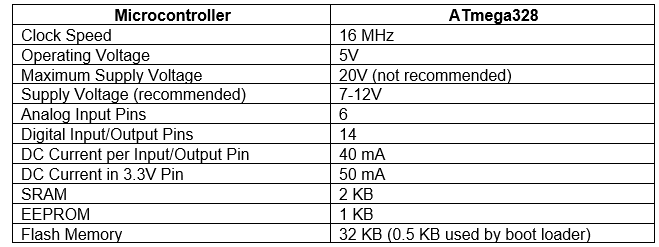
Pin Diagram of LED
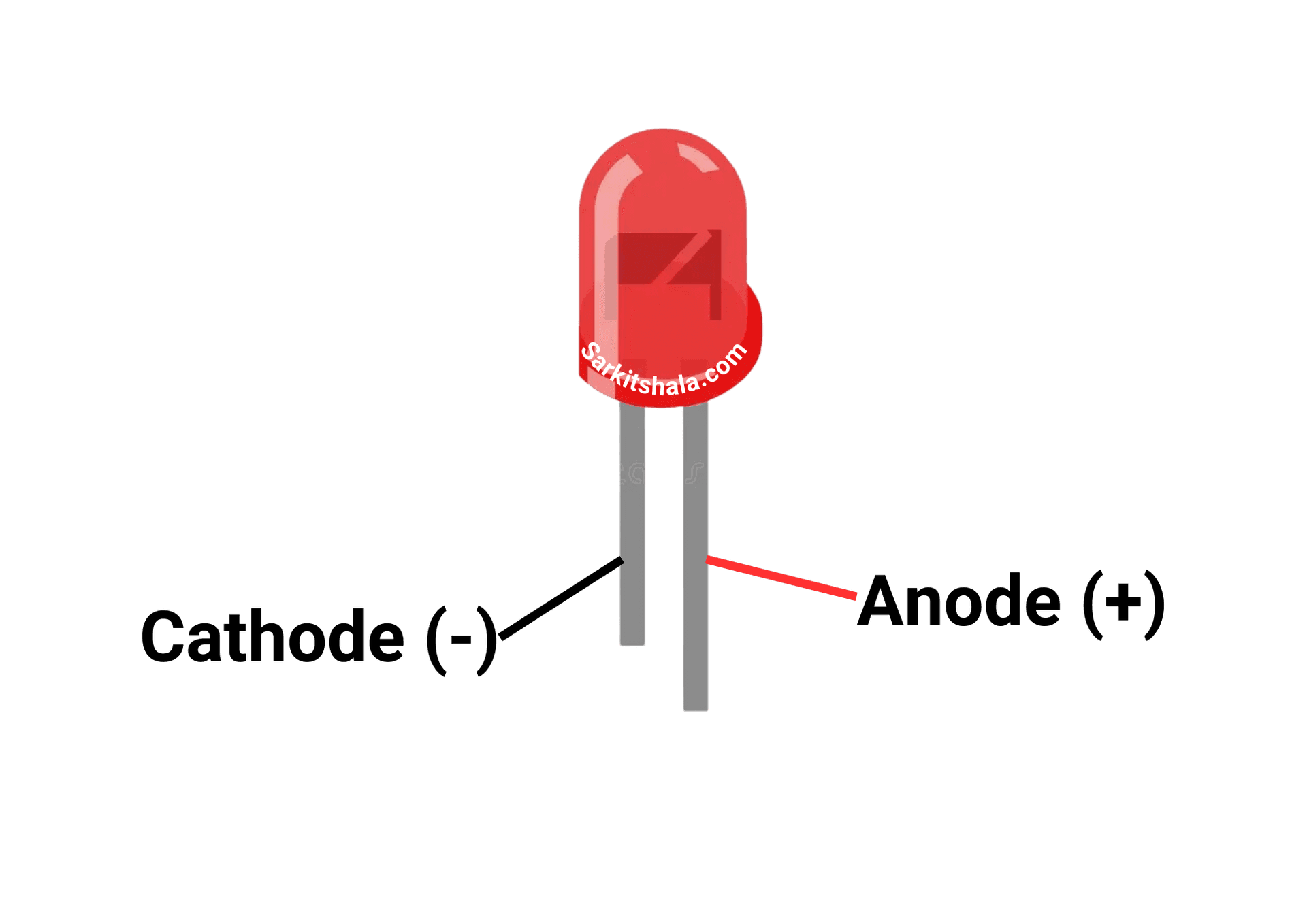
Circuit Diagram
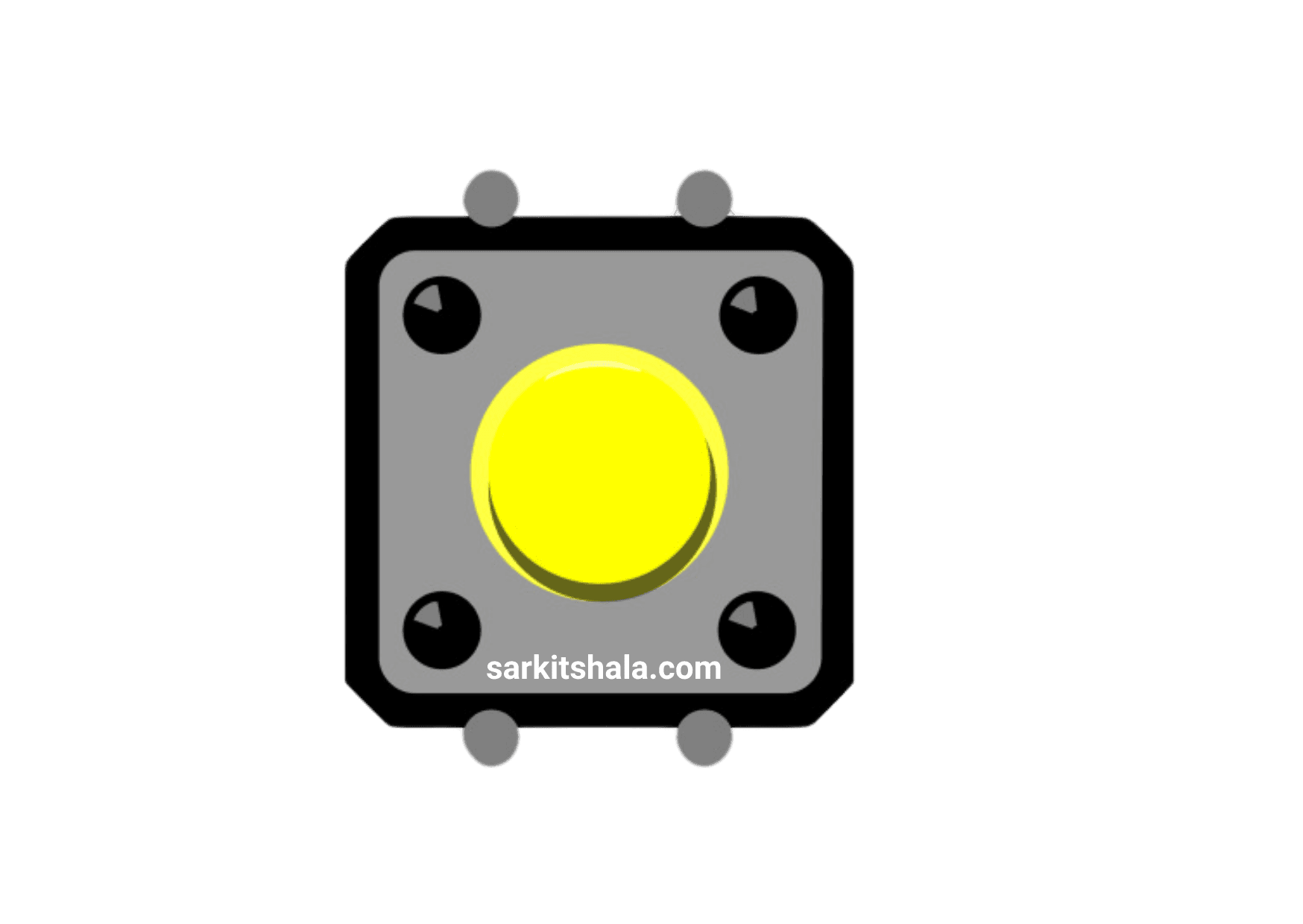
Steps
1. Connect LED with the Arduino Board.
2. Connect the Push Button with Arduino Board.
3. Connect the B-Type cable with the microcontroller.
4. Install and write code in Arduino IDE.
5. Compile and upload the code in Arduino .
Code
1
2#define LED_PIN 13 // Pin where the LED is connected
3#define BUTTON_PIN 2 // Pin where the push button is connected
4
5void setup() {
6 // Initialize the LED pin as an output:
7 pinMode(LED_PIN, OUTPUT);
8
9 // Initialize the BUTTON_PIN as an input with an internal pull-up resistor:
10 pinMode(BUTTON_PIN, INPUT_PULLUP);
11}
12
13void loop() {
14 // Read the state of the push button
15 int buttonState = digitalRead(BUTTON_PIN);
16
17 // Check if the button is pressed
18 if (buttonState == LOW) { // Button pressed (since we are using INPUT_PULLUP)
19 digitalWrite(LED_PIN, HIGH); // Turn the LED on
20 } else {
21 digitalWrite(LED_PIN, LOW); // Turn the LED off
22 }
23}
24
25