- LED Blink with Button
- Motion Detection Alarm System
- Simple RGB LED Controller
- Blinking LED with WebSocket Control
- Control LED Brightness using PWM
- Web Page with HTML and CSS on ESP32
- Control Multiple LEDs
- ESP32 with Bluetooth Serial Communication
- EEPROM with ESP32
- ESP32 Push Button Input: Reading Digital States
- Interfacing DHT11 Sensor
- Interfacing Ultrasonic Sensor
- Interfacing Flame Sensor
- Interfacing Sound Sensor
- Interfacing Potentiometer
- Interfacing IR Sensor
- Interfacing Servo Motor
- Interfacing Cam Wireless
- Interfacing DC Motor
- Interfacing Shock Sensor
- Interfacing Color Recognition Sensor
- Interfacing RFID Module
- TTGO LoRa32 SX1276 OLED
- Interfacing Keypad
- Interfacing Solenoid Lock
- Interfacing 16x2 LCD
- Interfacing Soil Moisture
- Interfacing MQ-7 Gas Sensor
- Interfacing Light Sleep Mode
- Interfacing Smart Light Control
- Interfacing (IoT) Weather Station
- Interfacing Web Server for Temperature Data Display
- Interfacing Home Automation System with Relay Control
- Interfacing IoT Smart Garden
- Face Recognition-Based Door Unlock System
- Interfacing Wi-Fi Jammer Detector
- Interfacing Health Band with Pulse
- Interfacing Sound Level Logger for Classrooms
- Night Vision Surveillance Camera
- Solar Panel Monitoring System
- Smart Farming Robot for Crop Surveillance
- Smart Water Quality Monitoring System
- Industrial IoT Gateway for Real-Time Monitoring
- Agriculture System with Automated Drone Control
Interfacing IR Sensor with ESP32
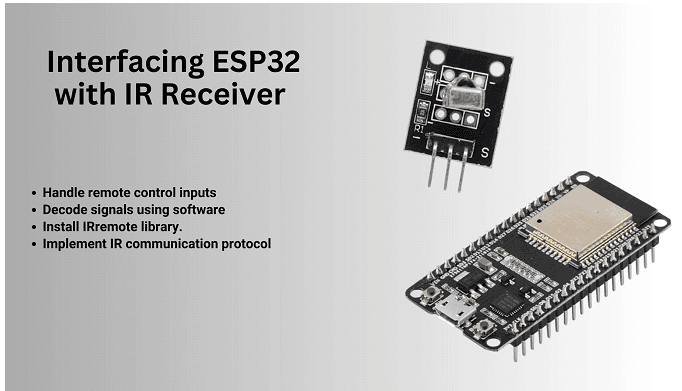
What is an IR Sensor?
An IR sensor, typically used for object detection and obstacle avoidance, works by emitting infrared light and measuring the reflection of that light. It is commonly used in robotics, home automation, and security systems.
Working Principle of IR Sensor
The IR sensor operates based on the reflection of infrared light:
- The IR LED emits infrared light.
- The infrared light hits an object and reflects back.
- The sensor detects the reflected light and produces a signal indicating the presence or absence of an object.
Formula: Object detected = (Reflected light intensity > Threshold)
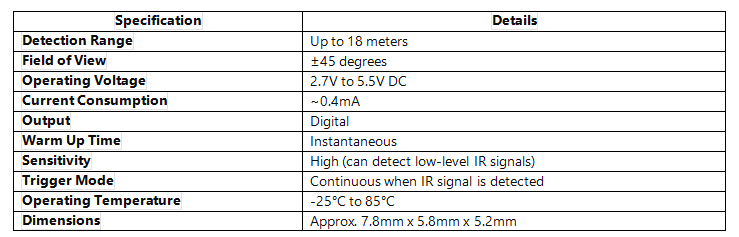
Components Required
- ESP32 board
- IR Sensor (e.g., TCS3200, KY-032)
- Jumper wires
- Breadboard (optional)
Pin Configuration of IR Sensor
- VCC: Power supply (typically +3.3V or +5V depending on sensor)
- GND: Ground
- OUT: Output pin (Digital signal indicating detection)
Ensure the IR sensor operates at 3.3V logic level for ESP32 compatibility.
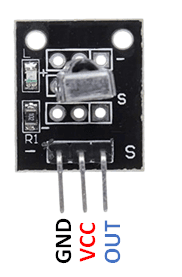
Wiring IR Sensor to ESP32
- VCC -> 3.3V (or 5V if supported)
- GND -> GND
- OUT -> GPIO 14
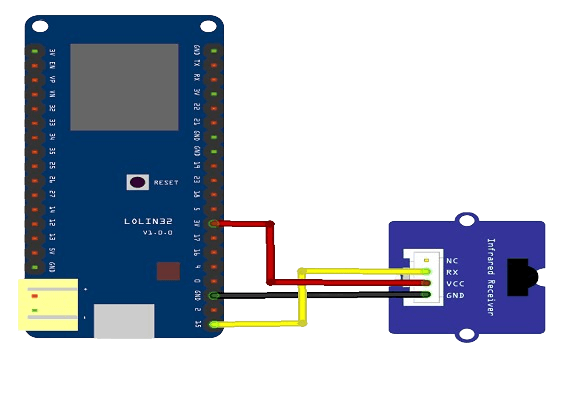
Arduino Code for ESP32 + IR Sensor
1#define IR_PIN 14
2
3int sensorValue;
4
5void setup() {
6 Serial.begin(115200);
7 pinMode(IR_PIN, INPUT);
8}
9
10void loop() {
11 sensorValue = digitalRead(IR_PIN);
12
13 if (sensorValue == HIGH) {
14 Serial.println("Object Detected");
15 } else {
16 Serial.println("No Object");
17 }
18
19 delay(500);
20}
Code Explanation (Line-by-Line)
- #define IR_PIN 14: Assigns GPIO 14 as the input pin connected to the IR sensor's output.
- Serial.begin(115200);: Starts serial communication for debugging/output.
- pinMode(IR_PIN, INPUT);: Sets the mode of IR_PIN as input to read the sensor's output.
- sensorValue = digitalRead(IR_PIN);: Reads the digital signal from the IR sensor. If the sensor detects an object, it returns HIGH, else LOW.
- if (sensorValue == HIGH) {...}: Checks if the sensor output is HIGH (object detected). If true, it prints 'Object Detected'.
- Serial.println("No Object");: If no object is detected (LOW), it prints 'No Object'.
Applications
- Obstacle Avoidance in Robots
- Object Detection for Automation Systems
- Line Following Robots
- Security Systems
- Home Automation (e.g., Automatic Doors)